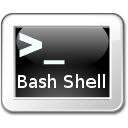
In this post we will see how to remove the newline character and combine two lines in a text file using "sed".
In sed, we have single line version and multi-line version of few commands. One such command is the next command. Click here to see an example for single-line version of next command in sed.
You might want to look at :
The processing space of the sed editor is known as the pattern space.
The multi-line next command (N) adds the next line to the existing
pattern space which already contains the previous line. So, the sed
editor can treat two lines as a single line. Below is an example for the N command.
The above sed editor script searches for the line of text that contains the word “first” in it. When it finds the line, it uses the N command to combine the next line with that line. It then uses the substitution command (s) to replace the newline character with a space. The result is that the two lines in the text file appear as one line in the sed editor output.
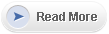